Building an Upsell offers Mini
What will you learn?
In this guide, you'll learn how to do the following:
- Set up an extension.
- Accept data from an order.
- Render product offer card on the orders screen.
Prerequisites
This guide assumes that you have your test store set up and that you have already been onboarded to the Minis Platform.
Step 1: Set up your mini's extension
When you created your mini, you would have been prompted to create an extension. For this guide, we want to use a specific extension called ProductOfferCard
, so if you chose to create a different one previously, you'd still need to create another one.
Run the shop-minis create-extension
command to create an extension. We'll choose the Order Management Page - After Order Details
target which already comes with a template using the ProductOfferCard
extension.
npx shop-minis create-extension
The ProductOfferCard
extension comes with a basic query input.graphql
that fetches the order's id. This data will be used by the mini.
Step 2: Reading data from the extension
In your mini you can see the template code using the useMinisParams()
hook to get an extensionData
object which contains data that the extension has fetched.
const {extensionData} = useMinisParams()
The useMinisParams
hook above doesn't yet have a type to gives typing to extensionData
. We can replace it with the type generated from the extension's input query.
import {OrderManagementOrderDetailsRenderAfterQueryData} from './targets/shop.order-management.order-details.render-after/input.graphql'
// ...
const {extensionData} = useMinisParams<OrderManagementOrderDetailsRenderAfterQueryData>()
Now we can access the order's ID from the query.
Step 3: Fetch discounted price from order metafield
Before we continue with this step, please follow the guide on metafields first. This step assumes that your orders have a metafield with the key of discounted-price
and the type money
.
To be able to query metafield data on an order, we'd need to create an order first. Use the Shop app to create an order on your test store and use the Admin API to add metafield data to the order.
Let's update our extension's query to fetch discounted price of the offered product.
query OrderConfirmationPageOrderDetailsRenderAfter {
order {
id
discountedPrice: metafield(namespace: "app--12345--foo", key: "discounted-price") {
value
}
}
}
Now lets start the mini dev server.
npm start
Navigate to the order list screen and open the order. We'll see the extension rendered with some stub data.
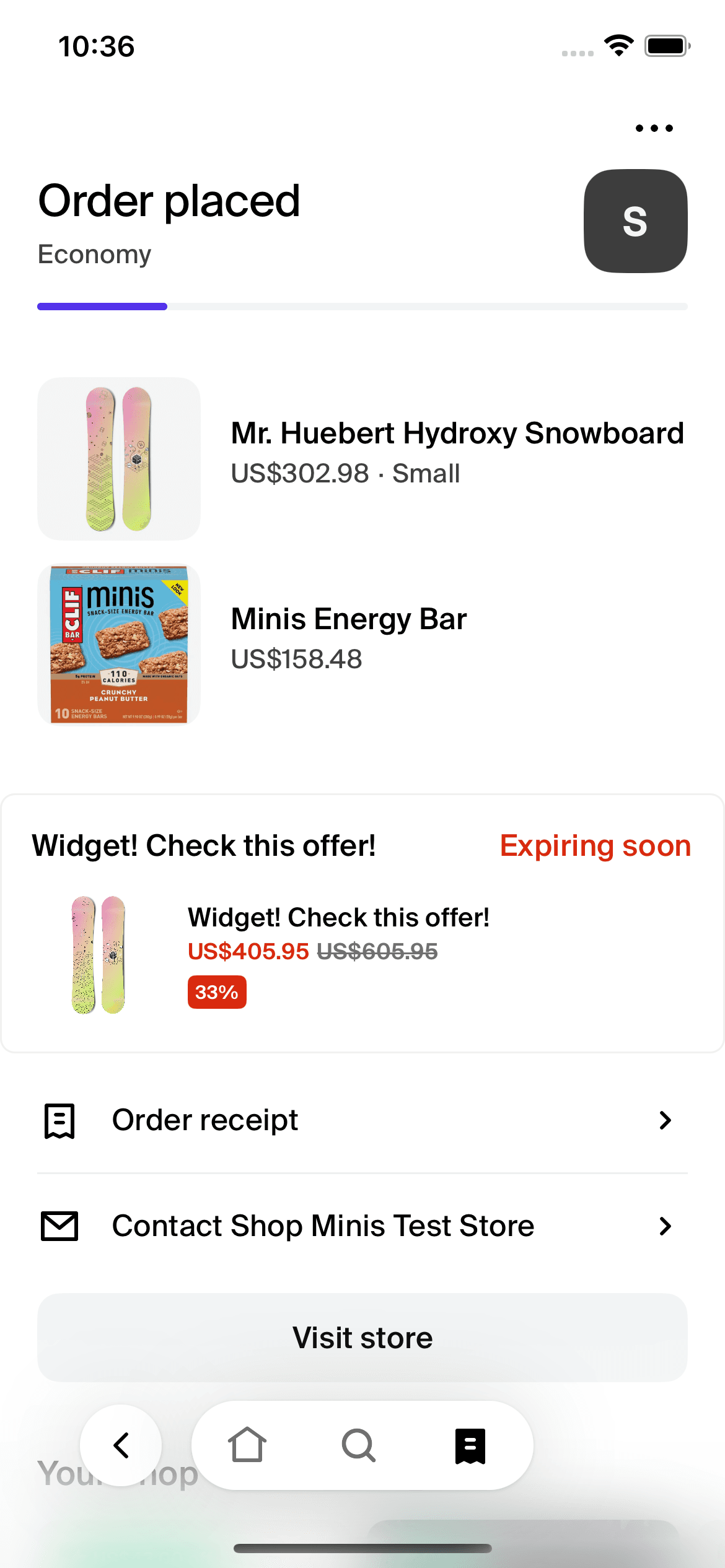
We'll discuss how the extension is rendered in the next step. For now, tap on the extension to open the mini and we'll see that the extensionData
now contains a stringified object of discounted price from the metafield.
Step 4: Customizing the product offer card extension
In the generated extension code inside the targets
directory we can see usage of a ProductOfferCard
component. The component is using stub data from a seed file.
Now that we are able to query custom data from the order metafield, we can explore the shape of the component props to see what data we have to provide to the component. For example, extensionData
now contains the discounted price from the metafield. This discounted price can be put in the offer
prop.
For the rest of the props, we can explore the available API and add more metafields if needed. Eventually, we should be able to replace the seed data with data from the query and / or other sources.
Step 5: Building your Upsell Offers mini
Post-purchase upsell offers can be very specific to your Shopify app. Please take advantage of our SDK components to build the UI required for your upsell flow.
We look forward to seeing what you build!