TouchableProduct
- The TouchableProduct component is a crucial interface element within Minis, especially in contexts where product interaction is required. This component encapsulates functionality for not only selecting a product but also navigating to detailed product pages with options like variant selection and discount application, as you can see used in the
ProductCardGrid
. It enhances the browsing experience in e-commerce apps by converting any of its child components into clickable elements that lead to more detailed product views.The functionality of
TouchableProduct
extends to managing navigation with specific product details such as variant IDs and discount codes, making it an integral part of the shopping experience. It leverages global actions and callbacks to handle these operations smoothly, ensuring a seamless user interface flow.Here’s how you can integrate the
TouchableProduct
into your React Native project: <TouchableProduct product={product}>
<ProductCard shopId={SHOP_GID} product={product} />
</TouchableProduct>
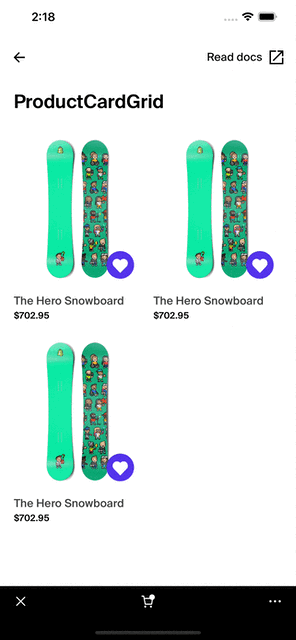
Requirements:
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
Integration
Step 1: Importing the Component
Start by importing the TouchableProduct
from your project's component library:
import { TouchableProduct } from '@shopify/shop-minis-platform-sdk';
Step 2: Using the TouchableProduct
Employ the TouchableProduct
within your application to wrap any product-related visual elements that should lead to a detailed view on press:
<TouchableProduct
product={someProductData}
onPress={() => navigateToProductDetailPage(someProductData.id)}
>
<ProductCard product={someProductData} />
</TouchableProduct>
Examples
Basic Usage:
<TouchableProduct
product={productDetails}
onPress={() => alert('Navigating to product details')}
>
<Text>Click to see more about this product!</Text>
</TouchableWebProduct>
With Product Variants and Discount Code:
<TouchableProduct
product={productDetails}
productVariantId="variant002"
discountCode="SUMMER_SALE"
onPress={() => handleProductSelection(productDetails.id)}
>
<ProductThumbnail product={productDetails} />
</TouchableProduct>
Props
- product (
ProductCardFragmentData | ProductLinkFragmentData
): The product data. - productVariantId (
string
, optional): Specifies the initially selected product variant. - includedProductVariantGIDs (
string[]
, optional): List of product variant GIDs to include. - discountCode (
string
, optional): Discount code to be applied at checkout. - attribution (
LineItemAttribution
, optional): Attribution metadata for tracking user actions. - onPress (
() => void
, optional): Callback function triggered when the product is pressed.
For more details on the props, please refer to the TouchableProduct Props.