ShareEmail
- The ShareEmail component is designed to facilitate the sharing of a user's email with a merchant within a React Native application, enhancing integration with e-commerce functionalities. This component prompts the user with a dialogue asking if they wish to share their email address, with the option to either comply or opt out ('Not now'). The response is handled via a callback function provided as a prop to the component.
This dialog component not only asks for email sharing but also offers to follow the store, which gives a dual functionality that is critical for engaging users and increasing store followers. It ensures that the user's desires are respected through clear communication and choices, thus fostering a trustworthy user experience.
Here’s an example of how to utilize the
ShareEmail
component: <ShareEmail
shopGID="shop_001"
onPress={(email) => {
if (email) {
console.log(`Email shared: ${email}`);
} else {
console.log("User chose not to share email.");
}
}}
/>
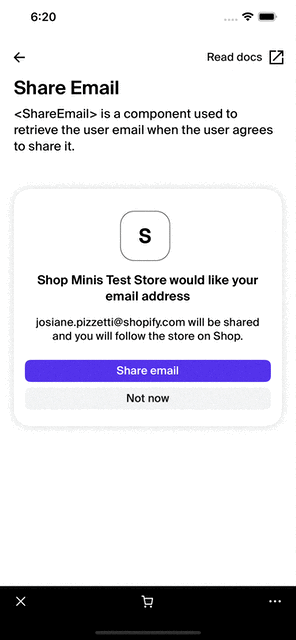
Integration
Requirements:
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
Step 1: Importing the Component
Import the ShareEmail
component from the @shopify/shop-minis-platform-sdk
pack:
import { ShareEmail } from '@shopify/shop-minis-platform-sdk';
Step 2: Implementing the ShareEmail
Incorporate the ShareEmail
in areas of your application that involve user interaction or are crucial for customer engagement:
<ShareEmail
shopGID="gid://shopify/Shop/12345"
onPress={(email) => console.log(email ? `Email shared: ${email}` : "Share declined")}
/>
Examples
Basic Prompt:
<ShareEmail
shopGID="gid://shopify/Shop/67890"
onPress={(email) => email ? console.log(`Thanks for sharing: ${email}`) : console.log("Maybe next time.")}
/>
With Default Email:
Use logic to pre-fill email using customer data if available:
<ShareEmail
shopGID="gid://shopify/Shop/12345"
onPress={(email) => handleEmailShare(email)}
/>
Props
- shopGID (
string
): The globally unique identifier for the shop. - onPress (
(email?: string | null) => void
): Callback function triggered when the user decides to share their email or opts out.
For more details on the props, please refer to the ShareEmail Props.