QuantityPicker
- The QuantityPicker component is an intuitive UI element specially designed for React Native applications that allows users to adjust quantities, typically for e-commerce platforms where customers select the number of items they wish to purchase. This component not only enhances user interaction but also provides essential functionality by allowing adjustments through increment and decrement buttons, and optionally, a delete button for removing an item or resetting the quantity.
Each interaction is carefully handled to ensure that the quantity remains within specified bounds, defined by minimum and maximum constraints. The component effectively manages focus states and user input, facilitating a seamless and error-free user experience.
Here's how to utilize the
QuantityPicker
component within your application: <QuantityPicker
quantity={1}
onQuantityChange={setQuantity}
minQuantity={1}
maxQuantity={10}
/>
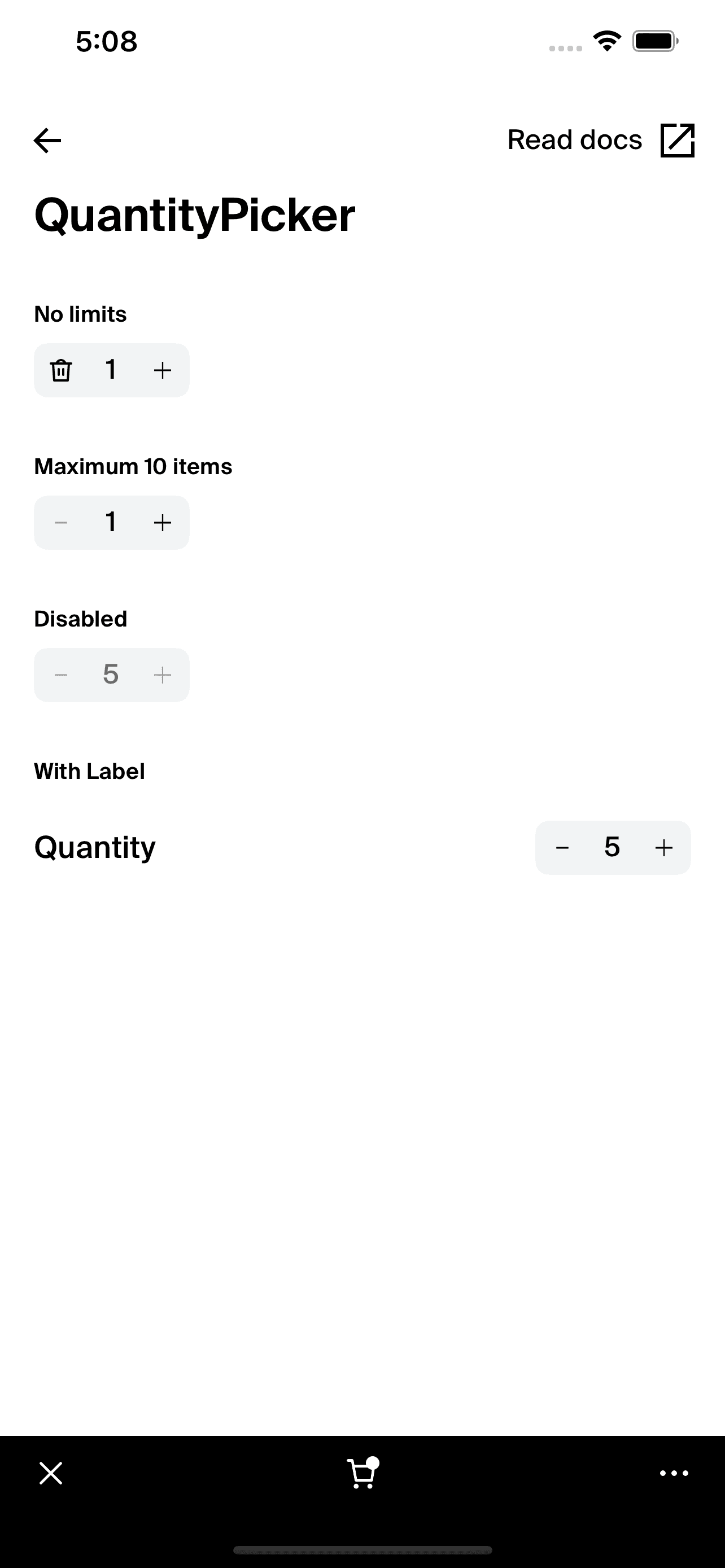
Requirements:
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
Integration
Step 1: Importing the Component
Import the QuantityPicker
from the @shopify/shop-minis-platform-sdk
:
import { QuantityPicker } from '@shopify/shop-minis-platform-sdk';
Step 2: Implementing the QuantityPicker
Implement the QuantityPicker
in your application wherever a numeric input is required for quantities, configuring it according to the min and max values required:
<QuantityPicker
quantity={productQuantity}
onQuantityChange={(newQuantity, isUserInteracted) => setProductQuantity(newQuantity)}
minQuantity={1}
maxQuantity={5}
label="Select quantity"
/>
Examples
Basic Use:
<QuantityPicker
quantity={2}
onQuantityChange={(newQuantity) => console.log(`Selected quantity: ${newQuantity}`)}
/>
With Min/Max Limits and Deletion Option:
<QuantityPicker
quantity={1}
minQuantity={1}
maxQuantity={20}
onQuantityChange={(qty) => console.log(`Adjusted to: ${qty}`)}
onDelete={() => console.log('Product removed from cart')}
/>
Props
- quantity (
number
): Current selected quantity. - onQuantityChange (
(newQuantity: number, userInteraction: boolean) => void
): Callback when the quantity changes. - minQuantity (
number
, optional): Minimum allowable quantity. - maxQuantity (
number
, optional): Maximum allowable quantity before the increment is disabled. - label (
string
, optional): Text label displayed next to the picker. - onDelete (
() => void
, optional): Callback function that is called when the quantity reaches its minimum and the delete option is exercised. - disabled (
boolean
, optional): Disables the quantity picker. - minMaxFeedbackEnabled (
boolean
, optional): Enables feedback when the quantity reaches the minimum or maximum limit. - flex (
number
, optional): Optionally allow the quantity picker to have flexible width.
For more details on the props, please refer to the QuantityPicker Props.