ProductLink
- The ProductLink component is meticulously designed for e-commerce platforms, enhancing product visibility while ensuring an interactive user experience. Incorporated within shop interfaces, it provides a swipeable, clickable display of products adapted to reflect user-favored states with seamless animations, impression tracking, and detailed product representation.
This component groups several features, including a thumbnail image, product title, variant price, and an optional favorites button. The integration of a fade-in animation on image load helps maintain a smooth user experience by subtly transitioning from a skeleton screen to the actual product image, raising engagement and reducing perceived wait times.
Here's how to integrate and utilize the
ProductLink
component within your Mini: <ProductLink
shopId="store123"
product={productData}
productVariantId={productData.variants.nodes[0].id}
onFavoriteToggled={(isFavorited) => toggleFavorite(isFavorited)}
/>
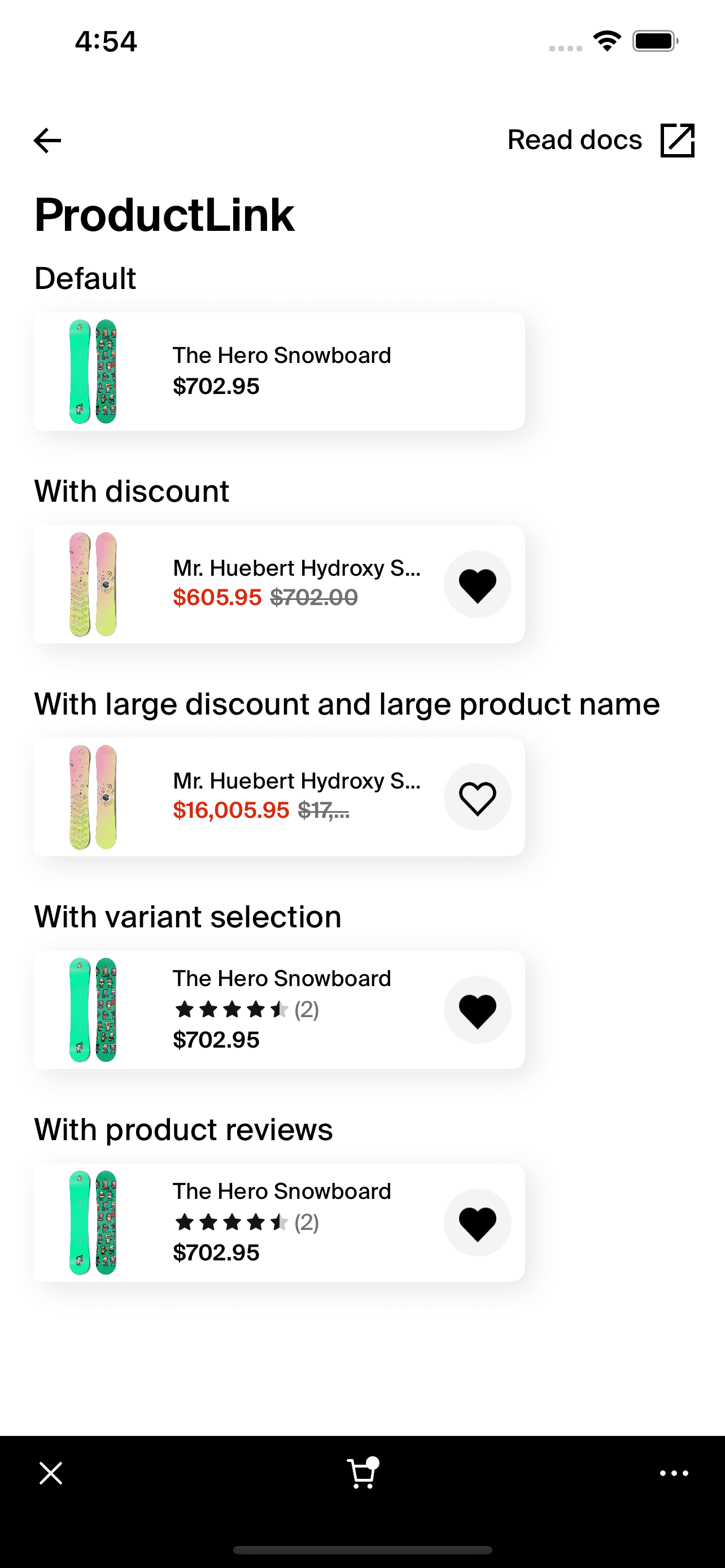
Integration
Requirements:
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
Step 1: Importing the Component
Start by importing the ProductLink
component from your components directory:
import { ProductLink } from '@shopify/shop-minis-platform-sdk';
Step 2: Using the ProductLink
Embed the ProductLink
in your shop or product list screens by providing necessary details about the product, shop, and variant:
<ProductLink
shopId="uniqueStoreID"
product={productData}
productVariantId={productData.variants.nodes[0].id}
sectionId="FeaturedProducts"
onPress={() => handlePress()}
onFavoriteToggled={(isFavorited) => handleFavoriteToggle(isFavorited)}
/>
Examples
Basic Usage of ProductLink:
<ProductLink
shopId="001"
product={sampleProduct}
productVariantId={sampleProduct.variants.nodes[0].id}
/>
Enhanced ProductLink with Event Handlers:
<ProductLink
shopId="002"
product={detailedProduct}
productVariantId={detailedProduct.variants.nodes[1].id}
onPress={() => alert('Product selected')}
onFavoriteToggled={(isFavorited) => toggleFavorite(isFavorited)}
/>
Props
- shopId (
string
, optional): The ID of the shop to which the product belongs. - product (
CustomProductLinkProps
): Detailed product object including title, variant, image, etc. - productVariantId (
string
, optional): Specific product variant ID to display. - sectionId (
string
, optional): Context or section where the product is being shown; used for tracking. - trackingDisabled (
boolean
, optional): Enable or disable impression tracking. - attribution (
LineItemAttribution
, optional): Attribution metadata for tracking user actions.- sourceName (
string
) - sourceIdentifier (
string
)
- sourceName (
- onFavoriteToggled (
(isFavorited: boolean) => void
, optional): Callback when the favorite status is toggled. - onPress (
() => void
, optional): Function to execute when the product is pressed.
For more details on the props, please refer to the ProductLink Props.