IconButton
caution
This documentation is outdated and only applies to partners with existing Minis. Please check back soon for new platform announcements.
- The IconButton component is a versatile user interface element designed for React Native applications that combines an icon display with touch functionality. It wraps an icon within a
TouchableOpacity
, allowing for various interactions. This component supports multiple variants including 'default', 'overlay', 'plain', and 'filled', each providing different visual styles.IconButton
is particularly useful for creating button controls that need to convey action through icons rather than text, such as a play button, settings gear, or any other symbolic actions. The optional blur effect on iOS for the 'overlay' variant enhances the aesthetic with a subtle background blur, adding a layer of depth and focus on the icon. <IconButton
name="heart"
onPress={() => console.log("Liked")}
variant="filled"
backgroundColor="red"
accessibilityLabel="Like"
/>
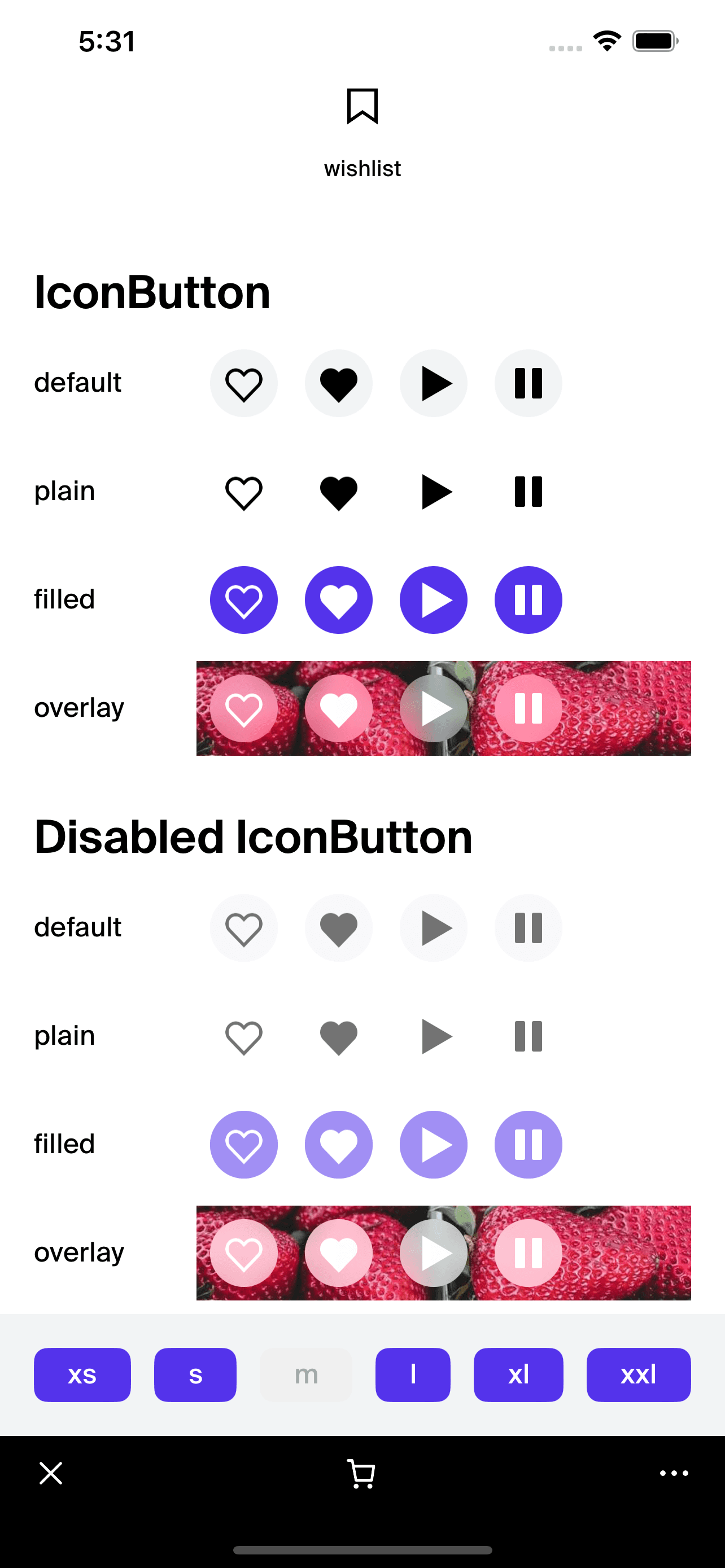
Integration
Requirements:
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
Step 1: Importing the Component
Make sure to import IconButton
in your component file:
import { IconButton } from '@shopify/shop-minis-platform-sdk';
Step 2: Using the IconButton Component
Implement the IconButton
by specifying the icon name
, onPress
function, and any desired variant
.
You can customize the appearance further with backgroundColor
and other accessible properties:
<IconButton
name="settings"
onPress={() => console.log("Settings pressed")}
variant="overlay"
accessibilityLabel="Open settings"
/>
Examples
Basic Usage of Default Variant:
<IconButton
name="search"
onPress={() => console.log("Searching")}
accessibilityLabel="Search"
/>
Filled Variant with Custom Background Color:
<IconButton
name="phone"
onPress={() => console.log("Calling")}
variant="filled"
backgroundColor="green"
accessibilityLabel="Make a call"
/>
Overlay Variant with iOS Blur Effect:
<IconButton
name="info"
onPress={() => console.log("Info")}
variant="overlay"
accessibilityLabel="Get information"
/>
The IconButton
component enhances UI interactivity by providing visually appealing and functional icon-based buttons. Its flexibility and easy customization make it suitable for a wide variety of applications, ensuring an intuitive and engaging user experience.
Additional Components
Icon
: Renders the icon within the button.Box
: Used for layout and styling of the button and the overlay.TouchableOpacity
: Provides the touch functionality.
Props
- name (
string
): The name of the icon to display (check the list of icons name through Icons demo - QR code). - onPress (
() => void
): The function to execute when the button is pressed. - variant (
IconButtonVariant
- required): The visual style of the button, 'default' | 'overlay' | 'plain' | 'filled'. - backgroundColor (
string
): The background color of the button. - accessibilityLabel (
string
): The label for accessibility purposes.
For more details on the props, please refer to the IconButton Props.