FavoritesButton
- The FavoritesButton component facilitates toggling the favorite status of products within a shopping environment, specifically engineered for applications leveraging Shopify platforms. It integrates seamlessly with GraphQL data structures to manage favorite states for individual product variants.
Using this component, users can easily mark products as favorites, enhancing their shopping experience by simplifying the way they track and return to products they are interested in. The component offers two stylistic variants, 'inline' and 'default', allowing it to integrate smoothly in various parts of the UI.
<FavoritesButton
shopId="shop_123"
productId="product_123"
productVariant={{
id: "variant_123",
isFavorited: false
}}
onFavoriteToggled={(isFavorited) =>
console.log("Favorite toggled:", isFavorited)
}
/>
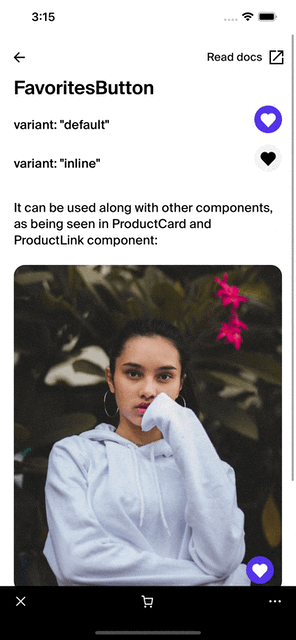
Integration
Requirements:
- Ensure your application harnesses React's context mechanisms and has access to GraphQL product variant data structures.
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
Step 1: Importing the Component
Import the FavoritesButton
at the start of your component file to ensure it is accessible within your project:
import { FavoritesButton } from '@shopify/shop-minis-platform-sdk';
Step 2: Using the FavoritesButton Component
Deploy FavoritesButton
in your application. Pass appropriate props such as shop and product identifiers, and handle changes via callbacks:
<FavoritesButton
shopId="62104633599"
productId="gid://shopify/ProductImage/31550124425261"
productVariant={{
id: "gid://shopify/ProductVariant/41471083544621",
isFavorited: true
}}
variant="inline"
onFavoriteToggled={(isFavorited) => console.log("Favorited status:", isFavorited)}
/>
Examples
Basic Usage:
<FavoritesButton
shopId="62104633599"
productId="gid://shopify/ProductImage/31550124425261"
productVariant={{
id: "gid://shopify/ProductVariant/41471083544621",
isFavorited: false
}}
/>
Inline Heart Icon Variant:
<FavoritesButton
shopId="62104633599"
productId="gid://shopify/ProductImage/31550124425261"
productVariant={{
id: "gid://shopify/ProductVariant/41471083544621",
title: "Product Title",
isFavorited: true
price: {
amount: 10.99,
currencyCode: "USD"
}
}}
variant="inline"
onFavoriteToggled={(isFavorited) => console.log("Toggled", isFavorited)}
/>
The usage of FavoritesButton
in a React Native application enables users to effortlessly manage their favorite products, improving interactivity and user experience in e-commerce platforms.
Additional Components
IconButton
: Utilized withinFavoritesButton
to provide the clickable heart icon.Box
: Provides layout and alignment for the heart icon by wrapping it.
Props
- shopId (
string
): The unique identifier for the shop. - productId (
string
): The unique identifier for the product. - variant (
"default" | "inline"
, optional): The stylistic variant of the button. - onFavoriteToggled (
(isFavorited: boolean) => void
, optional): Callback function triggered when the favorite status is toggled. - productVariant (
ProductVariant
): The product variant object containing the variant ID and favorite status.- id (
string
): The unique identifier for the product variant 'gid://shopify/ProductVariant/4147108354462'. - isFavorited (
boolean
): The favorite status of the product variant, true or false. - title (
string
, optional): The title of the product variant. - image (
Image
, optional): The image of the product variant. - price (
Price
): The price of the product variant. - compareAtPrice (
CompareAtPrice
, optional): The compare-at price of the product variant.
- id (
For more details on the props, please refer to the FavoritesButton Props.