Avatar
- The Avatar component is a versatile component designed to display a user profile image alongside optional text. The avatar can be customized extensively through various props to fit different use cases, such as in user profiles, comments sections, or anywhere user representation is needed.
The
Avatar
component typically consists of an image that represents the user, with an optional text title next to it. If no image is provided, a placeholder generated from the title is used instead, which helps maintain user identification intuitively and visually. <Avatar
title="John Doe"
source={{ uri: 'https://example.com/johndoe.jpg' }}
showTitle
size="medium"
color="foregrounds-regular"
/>
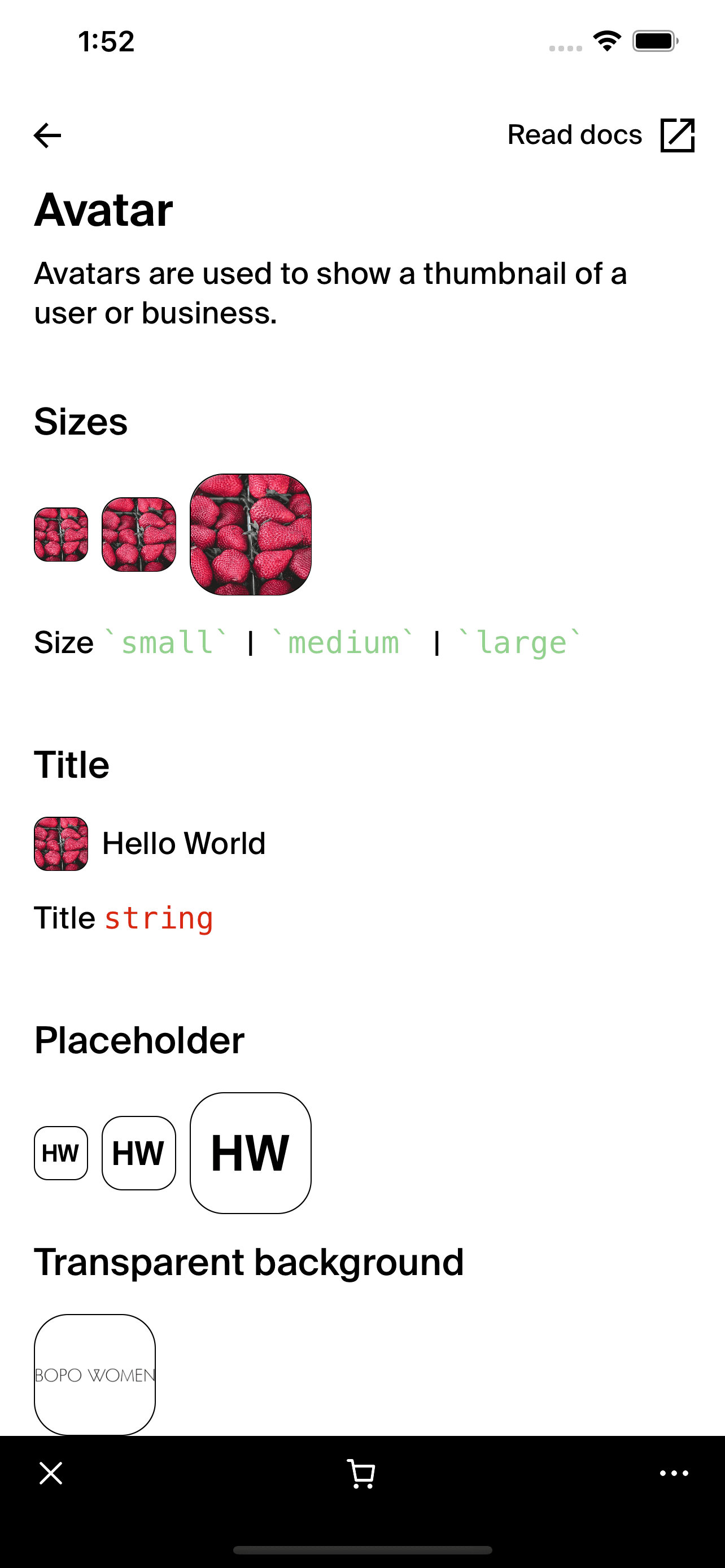
Integration
Requirements:
- Ensure your project environment is set up with access to
@shopify/shop-minis-platform-sdk
. See the quickstart for requirements and to set up a Shop Mini.
CDN Image Usage:
- For optimal performance, it is recommended to use images hosted on Shopify's CDN (e.g.,
https://cdn.shopify.com/s/files/1/0638/...
).
Step 1: Importing the Component
Firstly, ensure the Avatar
component is accessible by importing it at the top of your file:
import { Avatar } from '@shopify/shop-minis-platform-sdk';
Step 2: Using the Avatar
Component
Incorporate the Avatar
into your component structure by using it in the return statement of your component, providing all necessary props as needed:
<Avatar
title="Jane Doe"
source={{ uri: 'https://example.com/janedoe.jpg' }}
/>
Examples
Basic Usage:
<Avatar
title="John Doe"
source={{ uri: 'https://example.com/johndoe.jpg' }}
/>
With Title and Custom Color:
<Avatar
title="John Doe"
showTitle
source={{ uri: 'https://example.com/johndoe.jpg' }}
color="blue"
/>
Only Title Placeholder (No Image):
<Avatar
title="John Doe"
/>
Having an efficient and visually appealing Avatar
component is crucial for any application that emphasizes user interaction and identity.
By following these steps and examples, you can integrate and utilize the Avatar
component effectively in your app,
enhancing the user experience with customizable and interactive elements.
Additional Components
AvatarPlaceholder
: A sub-component that handles the rendering of the title abbreviation when no valid imageuri
is present.Text
,Box
,ImageBox
: Sub-components used for layout and styling purposes.
Props
- title (
string
): The text to display next to the avatar or used to generate the placeholder. - showTitle (
boolean
): Flag to control the visibility of the title next to the avatar. Defaults tofalse
. - source (
FastImageProps['source']
): The source of the avatar image. Accepts both URL and local image sources. - color (
Color
): Color for the avatar's border and title text. Defaults to 'foregrounds-regular'. - size (
AvatarSize
): Predefined sizes for the avatar, including 'small', 'medium', 'large', 'extra-large'. Defaults to 'small'.
For more details on the props, please refer to the Avatar Props.